# 刮刮乐
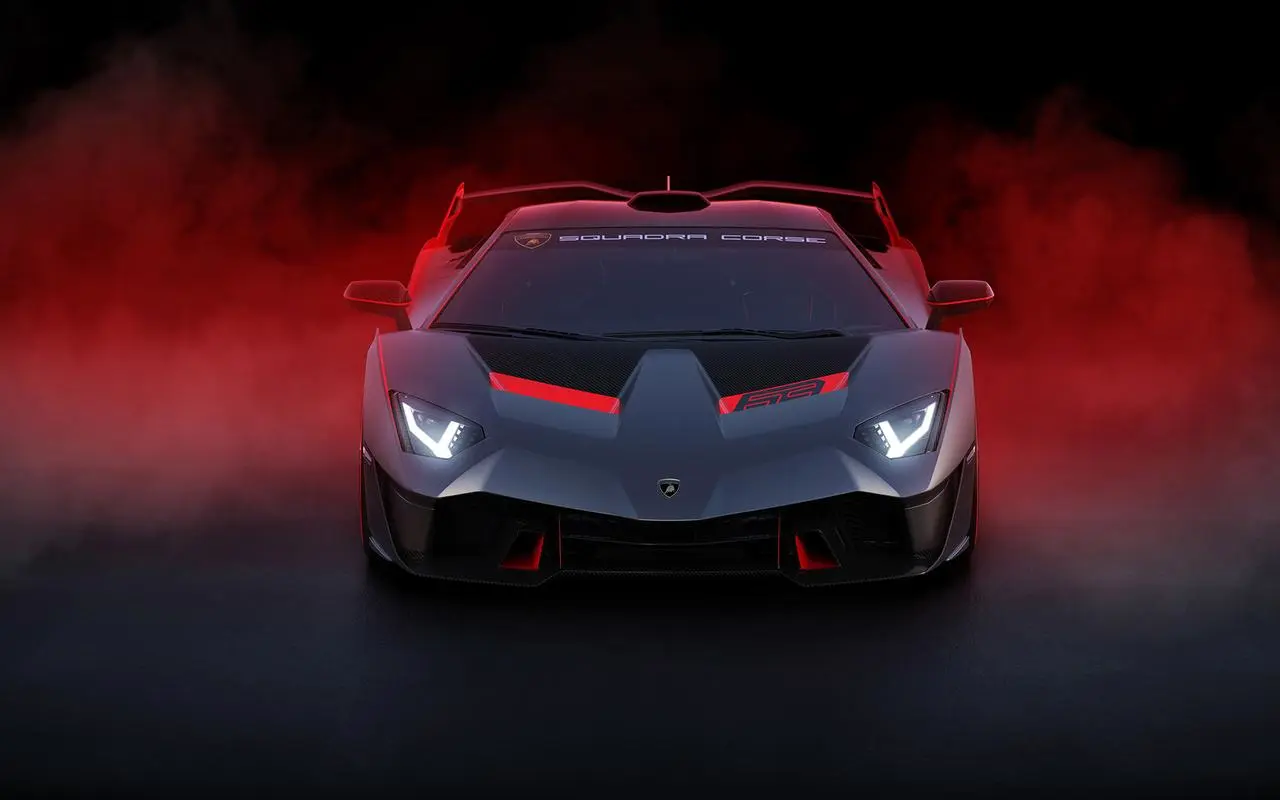
# canvas表格v0.0.1
(连简易版都不算,写着玩)
主要实现了下面这些功能
- 表格选中滚动
- 水平,垂直滚动条
- 滚动条手动控制滚动
- 边界处理(不允许越界)
计划支持以下特性
- 虚拟列表(满足不同浏览器尺寸的限制)
- 单元格可编辑
- 支持选中某行、某列或者全选
# 加速运动
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>加速运动</title>
<style>
#canvas {
border: 1px dashed #aaa;
}
</style>
</head>
<body>
<canvas id="canvas" width="1200" height="600"></canvas>
<script src="./ball.js"></script>
<script>
window.onload = () => {
const oCanvas = document.querySelector('#canvas')
const oGc = oCanvas.getContext('2d')
const width = oCanvas.width
const height = oCanvas.height
const ball = new Ball(0, height / 2)
let vx = 0;
let ax = 0.1;
(function linear() {
oGc.clearRect(0, 0, width, height)
ball.fill(oGc)
ball.x += vx
vx += ax
requestAnimationFrame(linear)
})()
}
</script>
</body>
</html>
# 加速运动分解与合成
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>加速运动分解与合成</title>
<style>
#canvas {
border: 1px dashed #aaa;
}
</style>
</head>
<body>
<canvas id="canvas" width="1200" height="600"></canvas>
<script src="./ball.js"></script>
<script>
window.onload = () => {
const oCanvas = document.querySelector('#canvas')
const oGc = oCanvas.getContext('2d')
const width = oCanvas.width
const height = oCanvas.height
const ball = new Ball(0, 0)
let a = 0.3,
ax = a * Math.cos(25 * Math.PI / 180),
ay = a * Math.sin(25 * Math.PI / 180),
vx = 0,
vy = 0;
(function linear() {
oGc.clearRect(0, 0, width, height)
ball.fill(oGc)
ball.x += vx
ball.y += vy
vx += ax
vy += ay
requestAnimationFrame(linear)
})()
}
</script>
</body>
</html>
# 抛物线运动
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>抛物线运动</title>
<style>
#canvas {
border: 1px dashed #aaa;
}
</style>
</head>
<body>
<canvas id="canvas" width="1200" height="600"></canvas>
<script src="./ball.js"></script>
<script>
window.onload = () => {
const oCanvas = document.querySelector('#canvas')
const oGc = oCanvas.getContext('2d')
const width = oCanvas.width
const height = oCanvas.height
const ball = new Ball(0, height / 2)
let vx = 2,
vy = -10,
gravity = 0.2;
(function linear() {
oGc.clearRect(0, 0, width, height)
ball.fill(oGc)
ball.x += vx
ball.y += vy
vy += gravity
if (ball.y >= height / 2) {
ball.y = height / 2
vy = -10
}
requestAnimationFrame(linear)
})()
}
</script>
</body>
</html>
# 重力弹跳
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>重力弹跳</title>
<style>
#canvas {
border: 1px dashed #aaa;
}
</style>
</head>
<body>
<canvas id="canvas" width="1200" height="600"></canvas>
<script src="./ball.js"></script>
<script>
window.onload = () => {
const oCanvas = document.querySelector('#canvas')
const oGc = oCanvas.getContext('2d')
const width = oCanvas.width
const height = oCanvas.height
const ball = new Ball(width / 2, 0)
let vy = 0,
gravity = 0.2,
bounce = -0.8
;(function linear() {
oGc.clearRect(0, 0, width, height)
ball.fill(oGc)
ball.y += vy
if (ball.y > canvas.height - ball.radius) {
ball.y = canvas.height - ball.radius
vy = vy * bounce
}
vy += gravity
requestAnimationFrame(linear)
})()
}
</script>
</body>
</html>
# 抛物线与重力弹跳运动
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>抛物线与重力弹跳运动</title>
<style>
#canvas {
border: 1px dashed #aaa;
}
</style>
</head>
<body>
<canvas id="canvas" width="1200" height="600"></canvas>
<script src="./ball.js"></script>
<script>
window.onload = () => {
const oCanvas = document.querySelector('#canvas')
const oGc = oCanvas.getContext('2d')
const width = oCanvas.width
const height = oCanvas.height
const ball = new Ball(0, height)
let vy = -10,
vx = 5,
gravity = 0.2,
bounce = -0.8
;(function linear() {
oGc.clearRect(0, 0, width, height)
ball.fill(oGc)
ball.x += vx
ball.y += vy
if (ball.y > canvas.height - ball.radius) {
ball.y = canvas.height - ball.radius
vy = vy * bounce
}
vy += gravity
requestAnimationFrame(linear)
})()
}
</script>
</body>
</html>
# 摩擦力运动
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>摩擦力运动</title>
<style>
#canvas {
border: 1px dashed #aaa;
}
</style>
</head>
<body>
<canvas id="canvas" width="1200" height="600"></canvas>
<script src="./ball.js"></script>
<script>
window.onload = () => {
const oCanvas = document.querySelector('#canvas')
const oGc = oCanvas.getContext('2d')
const width = oCanvas.width
const height = oCanvas.height
const ball = new Ball(0, height - 20)
let vx = 20,
friction = 0.98
;(function linear() {
oGc.clearRect(0, 0, width, height)
ball.fill(oGc)
ball.x += vx
vx *= friction
requestAnimationFrame(linear)
})()
}
</script>
</body>
</html>
css →